like this:
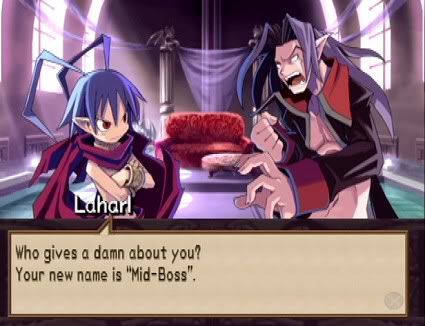
unfortunately it's not really working. The image is leaving a trial and the animation is a bit blocky. I thought I'd fix the blocky movement by adding more iterations to the animation loop, but my real problem is the trails it's leaving behind.
here's my code and these images need to be placed in the same folders as the EBOOT.PBP (if you wana see the problem in action)
http://i87.photobucket.com/albums/k134/ ... ground.png
http://i87.photobucket.com/albums/k134/hale550/left.png
Code: Select all
#include <pspdisplay.h>
#include <pspctrl.h>
#include <pspkernel.h>
#include <pspdebug.h>
#include <pspgu.h> //gives access to hardware acceleration also required by graphics.h
#include <png.h>
#include <stdio.h> //contains standard c functions
#include "graphics.h" //includes functions for loading and displaying images
#define printf pspDebugScreenPrintf
void scroll_from_left(Image*left_image);
PSP_MODULE_INFO("Sprite Test 4", 0, 1, 1);
/*setup callbacks to prevent psp from freezing or exiting at inappropirate times*/
/*----------------------------------------------------------------------------------------------------------------------------*/
/* Exit callback */
int exit_callback(int arg1, int arg2, void *common) {
sceKernelExitGame();
return 0;
}
/* Callback thread */
int CallbackThread(SceSize args, void *argp) {
int cbid;
cbid = sceKernelCreateCallback("Exit Callback", exit_callback, NULL);
sceKernelRegisterExitCallback(cbid);
sceKernelSleepThreadCB();
return 0;
}
/* Sets up the callback thread and returns its thread id */
int SetupCallbacks(void) {
int thid = 0;
thid = sceKernelCreateThread("update_thread", CallbackThread, 0x11, 0xFA0, 0, 0);
if(thid >= 0) {
sceKernelStartThread(thid, 0, 0);
}
return thid;
}
/*----------------------------------------------------------------------------------------------------------------------------*/
/*start the program*/
int main()
{
SceCtrlData pad; //declare pad of type "sceCtrlData"
char left_buffer[200]; //path to left image
char right_buffer[200]; //path to right image
char background_buffer[200]; //path to right image
Image*left; //create an a variable "left" of type Image in graphics.h
Image*right; //create an a variable "right" of type Image in graphics.h
Image*background;
//program setup
pspDebugScreenInit();
SetupCallbacks();
initGraphics(); //gets the PSP ready to display graphics; it does all of the initial setup.
sprintf(left_buffer, "left.png"); //print to a string the path to "left.png" in the variable "buffer"
sprintf(right_buffer, "right.png"); //print to a string the path to "right.png" in the variable "buffer"
sprintf(background_buffer, "background.png"); //print to a string the path to "background.png" in the variable "buffer"
left = loadImage(left_buffer); //define left image
right = loadImage(right_buffer); //define right image
background = loadImage(background_buffer); //define right image
sceDisplayWaitVblankStart(); //allows "home" button to work
sceCtrlReadBufferPositive(&pad, 1); //read from control pad input
blitAlphaImageToScreen(0 ,0 ,480 , 272, background, 0,0); //blit background
flipScreen; //flip background
scroll_from_left(left); //animate left.png
/*pause the program*/
sceKernelSleepThread();
return 0;
}
// scroll image from left to right
void scroll_from_left(Image*left_image)
{
int x_offset; //image offset from screen
int image_width; //png image width
int i; //counter
image_width = left_image->imageWidth; //find image width and store into "image_width"
x_offset = 0 - image_width; //determine what position on the screen the image should start out (not currently visible)
blitAlphaImageToScreen(0 ,0 ,image_width , 272, left_image , x_offset,0);
flipScreen();
int incriment = image_width/10; //divide the image width by the amount of frames (i) to define the incriment of movement
for(i=0;i<=10;i++) //animate image (scroll onto screen)
{
x_offset = x_offset + incriment;
pspDebugScreenClear(); //clear the screen
blitAlphaImageToScreen(0 ,0 ,image_width , 272, left_image, x_offset,0);
flipScreen();
sceKernelDelayThread(100000); //wait for 1/10 of a second
}
//position the image perfectly on the screen
pspDebugScreenClear();
blitAlphaImageToScreen(0 ,0 ,image_width , 272, left_image, 0,0);
flipScreen();
return;
}
Code: Select all
TARGET = sprite_practice4
OBJS = main.o graphics.o framebuffer.o
CFLAGS = -O2 -G0 -Wall
CXXFLAGS = $(CFLAGS) -fno-exceptions -fno-rtti
ASFLAGS = $(CFLAGS)
LIBDIR =
LIBS = -lpspgu -lpng -lz -lm
LDFLAGS =
EXTRA_TARGETS = EBOOT.PBP
PSP_EBOOT_TITLE = sprite_practice4
PSPSDK=$(shell psp-config --pspsdk-path)
include $(PSPSDK)/lib/build.mak